Working with accounts is one of the most crucial aspects of using the Partner Panel.
Account CRUD subsection covers methods related to CRUD (Create, Read, Update, Delete) operations for accounts. It includes:
- Creating accounts: Methods to create new accounts in the system.
- Deleting accounts: Methods to remove accounts from the system.
- Updating accounts: Methods to update existing account information.
- Retrieving account information: Methods to fetch details of specific accounts.
Account creation
Working with accounts is one of the most crucial aspects of using the Partner Panel.
There are several types of accounts available for creation and managing in the Partner Panel:
- Forguard accounts: Fully partner-managed accounts with access to the Forguard application. Partners who have completed full company verification have control over these accounts, including the ability to create and apply personalized tariff plans on client accounts. Without completing company verification, partners will not be able to use the Forguard solution.
- Ruhavik/Petovik accounts: These accounts have access to Ruhavik and (or) Petovik applications; however, they come with limitations on partner access after activation by clients.
When creating a client account, an application is assigned to the account, granting the client access.
This is implemented through a request, with one of the mandatory fields being "reg_apps".
IDs of applications:
- Ruhavik - 5a5ca87f-7cbe-4540-ab5d-77bf4bf69884
- Petovik - 962e19f0-6b4a-4f81-a3fe-4b657689b6f9
- Forguard - b901da51-ce00-4af2-b978-8d0fca8ae1ea
User entities are created simultaneously with the creation of a client account and are initially inactive. To activate the account, partner sends a special activation link to their client. The link format is the following:
https://login.gurtam.space/registration/acknowledgment?client_id=00010000000000000500&login=test_user_name&login_key=1123456
Where:
- client_id indicates the application affiliation:
- 00010000000000000500: Forguard
- 00010000000000000300: Ruhavik
- 00010000000000000400: Petovik
- test_user_name is replaced with the name of the user of this account
- login_key is the assigned login key during account creation.
Upon clicking the activation link, the client enters his/her unique email address (to be associated with the account) and a password. Then the client will receive a registration confirmation email at the provided address.
By following the link in the email, the client completes the registration process by entering the requested information in the form (which could include password, login key, etc.).
Once the client confirms registration, their account is activated, and the activation date and time are recorded in the 'ack' field. After that, the client will be able to log in to the application using the email and password that they entered during registration.
Create Ruhavik or Petovik account
To create a client account with access to the Ruhavik and/or Petovik applications, you should use POST method and send a request to the partner/accounts
endpoint:
POST /partner/accounts
The mandatory fields for this request are as follows:
- "reg_apps": [] - The application(s) ID to which the account will be granted access. An account can have simultaneous access to both the Ruhavik and Petovik applications, or only to one of them. In the example below, account is created with access to two applications.
IDs of applications:
- Ruhavik - 5a5ca87f-7cbe-4540-ab5d-77bf4bf69884
- Petovik - 962e19f0-6b4a-4f81-a3fe-4b657689b6f9
- "name": string - Unique user name. Length must be between 4 and 50.
- "login_key": string - Key for the client's initial login. Length must be between 4 and 50
In the request, the fields “title” and “description” are optional.
- “title”: string - Name of the account entity, is optional, in case it is not set ID of the account would be used as title. Length must be between 4 and 50
- “description”: string - Additional description or information about the account
Example of Request:
curl -X 'POST' \ 'https://api.gps-trace.com/partner/accounts' \
-H 'accept: application/json' \
-H 'X-AccessToken: <your token>' \
-H 'Content-Type: application/json' \
-d '{ "title": "First test account", "description": "First account description", "reg_apps": [ "5a5ca87f-7cbe-4540-ab5d-77bf4bf69884", "962e19f0-6b4a-4f81-a3fe-4b657689b6f9" ], "user": { "name": "test_user", "description": "user description", "login_key": "654sfd32Rf1w" } }'
Example of success response:
{
"data": {
"id": number,
"pid": number,
"title": "First test account",
"description": "First account description",
"link_app": null,
"reg_apps": [
"5a5ca87f-7cbe-4540-ab5d-77bf4bf69884",
"962e19f0-6b4a-4f81-a3fe-4b657689b6f9"
],
"created_at": number,
"updated_at": number,
"ack": number,
"subscriptions": {},
"service_apps": [],
"is_attached": false,
"attached_at": null,
"added_time": number,
"is_premium": false,
"type": null,
"tariff_plans": {},
"blocked": false,
"blocked_at": null,
"user": {
"id": "string",
"account_id": number,
"name": "test_user",
"email": null,
"description": "user description",
"login_key": null,
"lang": null
}
}
}
- id: The unique identifier assigned to the newly created account.
- pid: The partner account ID associated with the account.
- title: The name/title of the account, typically provided during creation. This field is optional, and if left unspecified during the creation process, the system will default to using the account's ID as its title.
- description: A description or additional information about the account, if provided during creation.
- link_app: The application ID upon initial self-registration.
- reg_apps: An array containing the IDs of the applications associated with the account. This indicates which applications the account has access to.
- created_at: Timestamp indicating the date and time when the account was created.
- updated_at: Timestamp indicating the date and time when the account was last updated.
- ack: Account activation date, represented as a timestamp.
- subscriptions: Object containing details about subscription associated with the account.
- service_apps: An array containing the applications associated with the account in which the service mode is enabled.
- is_attached: Boolean value indicating whether the account is linked by a partner.
- attached_at: If applicable, this field represents the date when the account was linked.
- added_time: Timestamp indicating the date and time when the account was added.
- is_premium: Boolean value indicating the active premium status subscription of the account.
- type: The type of the account:
- 10: Managed by partner account
- 20: Partner account
- null: Ruhavik/Petovik account
- tariff_plans: Object containing details about the client plan of the account, specifically related to Forguard.
- blocked: Boolean value indicating whether the account is currently blocked.
- blocked_at: This field indicates the date when the account will be automatically blocked.
- user:
- id: The unique identifier of the user.
- account_id: The ID of the account to which the user belongs.
- name: The name of the user.
- email: The email address of the user. Assigned after account activation by the client
- description: Additional description or information about the user.
- login_key: If applicable, the activation confirmation key for the user's account.
- lang: The language of the user.
Create Forguard account
To create a client's account with access to the Forguard app, you should use the following endpoint:
POST /partner/accounts/managed
When creating Forguard account, it is mandatory to assign a client plan. If there are no existing client' plans associated with the partner account, the first step is to create one using the POST partner/client-plans endpoint. For more detailed information, please see the Client plans management section.
Scheme for creating a Forguard account:
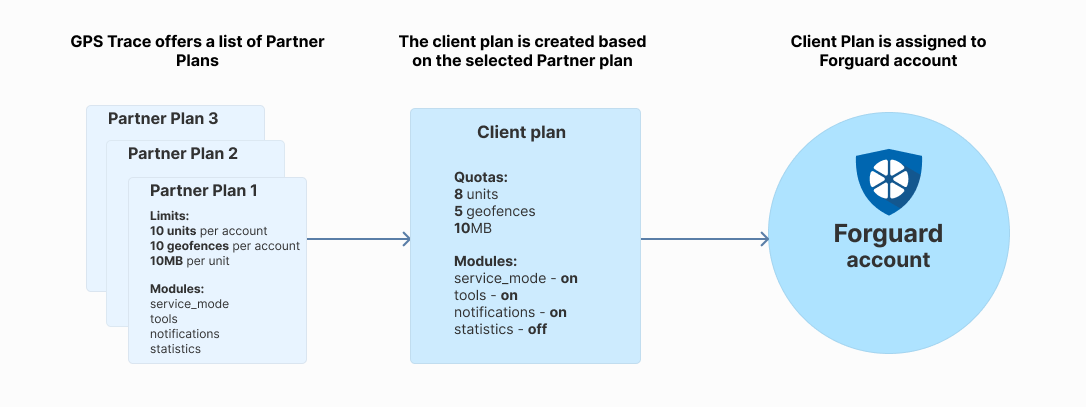
Required fields for this request are the following:
- reg_apps: [] - the list of application IDs to which the account will be granted access.
- Id of the Forguard app - b901da51-ce00-4af2-b978-8d0fca8ae1ea
- tariff_plans - this is an object in which key is an application ID and value is the ID of the client's plan. To retrieve detailed information about the client plans, you can make a request to the GET /partner/client-plans endpoint.
- name: string - unique name for the user. Length must be between 4 and 50.
- login_key: string - key for the client's initial login. Length must be between 4 and 50
The fields “title” and “description” are optional in this request.
- title: string - name of the account entity, is optional, in case it is not set ID of the account would be used as title. Length must be between 4 and 50
- description: string - additional description or information about the account
Example of Request:
curl -X 'POST' \
'https://api.gps-trace.com/partner/accounts/managed' \
-H 'accept: application/json' \
-H 'X-AccessToken: <your token>' \
-H 'Content-Type: application/json' \
-d '{
"title": "Forguard account",
"description": "Account description",
"reg_apps": [
"b901da51-ce00-4af2-b978-8d0fca8ae1ea"
],
"tariff_plans": {
"b901da51-ce00-4af2-b978-8d0fca8ae1ea": "string"
},
"user": {
"name": "test_user",
"login_key": "123Abc456"
}
}'
The response contains the same fields as the response for creating a Ruhavik/Petovik account, along with an additional field “tariff_plans” indicating the client plan assigned to the Forguard account.
Example of success response:
{
"data": {
"id": number,
"pid": number,
"title": "Forguard account",
"description": "Account descriptiont",
"link_app": null,
"reg_apps": [
"b901da51-ce00-4af2-b978-8d0fca8ae1ea"
],
"created_at": number,
"updated_at": number,
"ack": 0,
"subscriptions": {},
"service_apps": [],
"is_attached": false,
"attached_at": null,
"added_time": number,
"is_premium": false,
"type": 10,
"tariff_plans": {
"b901da51-ce00-4af2-b978-8d0fca8ae1ea": "string"
},
"blocked": false,
"blocked_at": null,
"user": {
"id": "string",
"account_id": number,
"name": "test_user",
"email": null,
"description": null,
"login_key": null,
"lang": null,
}
}
}
- id: unique identifier assigned to the newly created account.
- pid: partner account ID associated with the account.
- title: name/title of the account, typically provided during creation. This field is optional, and if left unspecified during the creation process, the system will default to using the account's ID as its title.
- description: additional information about the account, if provided during creation.
- link_app: application ID upon initial self-registration.
- reg_apps: an array containing the IDs of the applications associated with the account. This indicates which applications the account has access to.
- created_at: timestamp indicating the date and time when the account was created.
- updated_at: timestamp indicating the date and time when the account was last updated.
- ack: account activation date, represented as a timestamp.
- subscriptions: object containing details about subscription associated with the account.
- service_apps: an array containing the applications associated with the account in which the service mode is enabled.
- is_attached: boolean value indicating whether the account is linked by a partner.
- attached_at: if applicable, this field represents the date when the account was linked.
- added_time: timestamp indicating the date and time when the account was added.
- is_premium: boolean value indicating the active premium status subscription of the account.
- type: type of the account:
- 10 - managed by partner account (Forguard)
- 20 - partner account
- null - Ruhavik/Petovik account
- tariff_plans: object containing details about the client plan of the account, specifically related to Forguard. (“app ID”:”client plan ID”)
- blocked: boolean value indicating whether the account is currently blocked.
- blocked_at: field indicates the date when the account will be automatically blocked.
- user:
- id: unique identifier of the user.
- account_id: the ID of the account to which the user belongs.
- enabled: boolean value indicating whether the user has activated their account.
- name: the name of the user.
- email: the email address of the user. Assigned after account activation by the client
- description: additional description or information about the user.
- login_key: if applicable, the activation confirmation key for the user's account.
- lang: the language of the user.
Get accounts list
This method is used to retrieve a list of client accounts. It supports additional filtering by application_id of accounts IDs. Also you can optimize your call by requesting only the desired fields.
GET /partner/accounts
Example of request to retrieve all Forguard accounts using filtering.
curl -X 'GET' \ 'https://api.gps-trace.com/partner/accounts?app_id=b901da51-ce00-4af2-b978-8d0fca8ae1ea' \
-H 'accept: application/json' \
-H 'X-AccessToken: <your token>'
Example of success response:
"data": [
{
"id": number,
"pid": number,
"title": "string",
"description": "string",
"link_app": null,
"reg_apps": [
"b901da51-ce00-4af2-b978-8d0fca8ae1ea"
],
"created_at": number,
"updated_at": number,
"ack": number,
"subscriptions": {},
"service_apps": [],
"is_attached": false,
"attached_at": null,
"added_time": number,
"is_premium": false,
"type": 10,
"tariff_plans": {
"b901da51-ce00-4af2-b978-8d0fca8ae1ea": "string"
},
"blocked": false,
"blocked_at": number
}
]
- id: unique identifier of the account.
- pid: partner account ID associated with the account.
- title: name/title of the account
- description: description or additional information about the account
- link_app: application ID upon initial self-registration.
- reg_apps: an array containing the IDs of the applications associated with the account. This indicates which applications the account has access to.
- created_at: timestamp indicating the date and time when the account was created.
- updated_at: timestamp indicating the date and time when the account was last updated.
- ack: account activation date, represented as a timestamp.
- subscriptions: object containing details about subscription associated with the account.
- service_apps: array containing the applications associated with the account in which the service mode is enabled.
- is_attached: boolean value indicating whether the account is linked by a partner.
- attached_at: if applicable, this field represents the date when the account was linked.
- added_time: timestamp indicating the date and time when the account was added.
- is_premium: boolean value indicating the active premium status subscription of the account.
- type: type of the account:
- 10 - managed by partner account (Forguard)
- 20 - partner account
- null - Ruhavik/Petovik account
- tariff_plans: object containing details about the client plan of the account, specifically related to Forguard. (“app ID”:”client plan ID”)
- blocked: boolean value indicating whether the account is currently blocked.
- blocked_at: this field indicates the date when the account will be automatically blocked.
Get account by ID
This method is used to retrieve a client account by ID.
GET /partner/accounts/{id}
Request body example:
curl -X 'GET' \ 'https://api.gps-trace.com/partner/accounts/100100' \
-H 'accept: application/json' \
-H 'X-AccessToken: <your token>'
Example of success response
{
"data": {
"id": 100100,
"pid": number,
"title": "string",
"description": "string",
"link_app": null,
"reg_apps": [
"b901da51-ce00-4af2-b978-8d0fca8ae1ea"
],
"created_at": number,
"updated_at": number,
"ack": number,
"subscriptions": {},
"service_apps": [],
"is_attached": false,
"attached_at": null,
"added_time": number,
"is_premium": false,
"type": 10,
"tariff_plans": {
"b901da51-ce00-4af2-b978-8d0fca8ae1ea": "string"
},
"blocked": false,
"blocked_at": number
}
}
- id: unique identifier of the account.
- pid: partner account ID associated with the account.
- title: the name/title of account
- description: additional information about the account
- link_app: application ID upon initial self-registration.
- reg_apps: an array containing the IDs of the applications associated with the account. This indicates which applications the account has access to.
- created_at: timestamp indicating the date and time when the account was created.
- updated_at: timestamp indicating the date and time when the account was last updated.
- ack: account activation date, represented as a timestamp.
- subscriptions: object containing details about subscription associated with the account.
- service_apps: an array containing the applications associated with the account in which the service mode is enabled.
- is_attached: boolean value indicating whether the account is linked by a partner.
- attached_at: if applicable, this field represents the date when the account was linked.
- added_time: timestamp indicating the date and time when the account was added.
- is_premium: boolean value indicating the active premium status subscription of the account.
- type: type of the account:
- 10: managed by partner account (Forguard)
- 20: partner account
- null: Ruhavik/Petovik account
- tariff_plans: object containing details about the client plan of the account, specifically related to Forguard. (“app ID”:”client plan ID”)
- blocked: boolean value indicating whether the account is currently blocked.
- blocked_at: this field indicates the date when the account will be automatically blocked.
Update account
Use the PATCH method to update account details such as title, description, and the list of applications the account is associated with:
PATCH /partner/accounts/{id}
Please note, that if account belongs to Forguard, it cannot be changed to Ruhavik or vice versa. However, you can modify the association between Ruhavik and Petovik. In other words, if account has access to Ruhavik application, you can add access to Petovik or simply switch to Petovik. For updating user information, use a different method PATCH /partner/users/{id}.
"reg_apps" or in other words the application IDs to which the account will be granted access
Request example:
curl -X 'PATCH' \
'https://api.gps-trace.com/partner/accounts/101111' \
-H 'accept: application/json' \
-H 'X-AccessToken: <your token> ' \
-H 'Content-Type: application/json' \
-d '{
"title": "Account title",
"description": "Account description",
"reg_apps": [
"5a5ca87f-7cbe-4540-ab5d-77bf4bf69884",
"962e19f0-6b4a-4f81-a3fe-4b657689b6f9"
]
}'
Example of success response
{
"data": {
"id": 101111,
"pid": number,
"title": "Account title",
"description": "Account description",
"link_app": null,
"reg_apps": [
"5a5ca87f-7cbe-4540-ab5d-77bf4bf69884",
"962e19f0-6b4a-4f81-a3fe-4b657689b6f9"
],
"created_at": number,
"updated_at": number,
"ack": 0,
"subscriptions": {
"5a5ca87f-7cbe-4540-ab5d-77bf4bf69884": "td-basic.monthly"
},
"service_apps": [],
"is_attached": false,
"attached_at": null,
"added_time": number,
"is_premium": true,
"type": null,
"tariff_plans": null,
"blocked": false,
"blocked_at": null
}
}
- id: unique identifier of the account.
- pid: partner account ID associated with the account.
- title: name/title of the account.
- description: description or additional information about the account.
- link_app: application ID upon initial self-registration.
- reg_apps: array containing the IDs of the applications associated with the account. This indicates which applications the account has access to. IDs of applications:
- Forguard - b901da51-ce00-4af2-b978-8d0fca8ae1ea
- Ruhavik - 5a5ca87f-7cbe-4540-ab5d-77bf4bf69884
- Petovik - 962e19f0-6b4a-4f81-a3fe-4b657689b6f9
- created_at: timestamp indicating the date and time when the account was created.
- updated_at: timestamp indicating the date and time when the account was last updated.
- ack: account activation date, represented as a timestamp.
- subscriptions: object containing details about subscription associated with the account.
- service_apps: array containing the applications associated with the account in which the service mode is enabled.
- is_attached: boolean value indicating whether the account is linked by a partner.
- attached_at: if applicable, this field represents the date when the account was linked.
- added_time: timestamp indicating the date and time when the account was added.
- is_premium: boolean value indicating the active premium status subscription of the account.
- type: type of the account:
- 10: Managed by partner account
- 20: Partner account
- null: Ruhavik/Petovik account
- tariff_plans: Object containing details about the client plan of the account, specifically related to Forguard.
- blocked: Boolean value indicating whether the account is currently blocked.
- blocked_at: This field indicates the date when the account will be automatically blocked.
Delete account
Account deletion is performed using the following DELETE request:
DELETE /partner/accounts/{id}
Where id is unique ID of the account you want to remove.
It is important to note that Ruhavik/Petovik accounts, once activated by the client, become fully owned by the client. Therefore, partners cannot delete activated Ruhavik/Petovik accounts. This also applies to inactive Ruhavik accounts with an active Premium subscription - partners cannot delete such accounts.
On the other hand, Forguard accounts are fully managed by the partner and do not have any restrictions on actions from the partner's side.
Request body example:
curl -X 'DELETE' \
'https://api.gps-trace.com/partner/accounts/111111' \
-H 'accept: application/json' \
-H 'X-AccessToken: <your token>'
Upon successful deletion, a response with status code 200 will be returned, along with the ID of the removed account.